Java Method Server: A Detailed Overview
- waseem7130
- Mar 17
- 3 min read
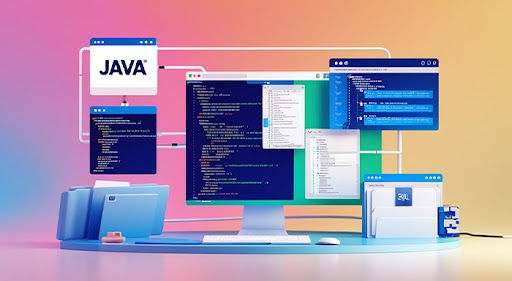
Enterprise applications require Java Method Server (JMS) in order for Java methods to run effectively on a dedicated server. By outsourcing intricate calculations and managing method execution in an organized way, it improves application speed. We will go into great detail about Java Method Server in this blog, outlining its features, advantages, and methodical implementation.
What is Java Method Server?
An specialized server that enables Java methods to be executed remotely or in a dedicated server environment is called a Java Method Server. Enterprise applications that demand great performance, scalability, and effective resource management will find it very helpful.
Key Features of Java Method Server
Remote Method Invocation (RMI): Enables execution of Java methods on a remote server, reducing the load on the client system.
Thread Management: Efficiently manages multiple method execution requests to optimize system performance.
Scalability: Supports handling of numerous client requests without impacting application efficiency.
Security: Provides access control and authentication mechanisms to safeguard method execution.
Logging and Monitoring: Facilitates logging of method execution details and real-time monitoring for performance optimization.
Load Balancing: Distributes execution requests evenly across available resources to prevent server overload.
Why Use Java Method Server?
Performance Enhancement: Offloads intensive computations from the client, ensuring smooth operation.
Resource Optimization: Utilizes server resources efficiently, preventing application lag.
Better Maintainability: Centralized execution logic makes debugging and updates easier.
Supports Distributed Computing: Ideal for applications requiring distributed processing of Java methods.
Improves Application Scalability: Allows applications to handle more requests without slowing down.
How Java Method Server Works
The following stages can be used to summarize how Java Method Server operates:
Client Request: A client application sends a request to execute a specific Java method.
Request Processing: The server receives and authenticates the request.
Method Execution: The requested Java method is executed on the server.
Response Handling: The server processes and returns the result to the client.
Logging and Monitoring: Execution details are logged for future analysis.
Implementing Java Method Server
To implement a simple Java Method Server, follow these steps:
Step 1: Define the Remote Interface
import java.rmi.Remote;
import java.rmi.RemoteException;
public interface MethodServer extends Remote {
String executeMethod(String input) throws RemoteException;
}
Step 2: Implement the Server
import java.rmi.server.UnicastRemoteObject;
import java.rmi.RemoteException;
public class MethodServerImpl extends UnicastRemoteObject implements MethodServer {
protected MethodServerImpl() throws RemoteException {
super();
}
public String executeMethod(String input) throws RemoteException {
return "Processed: " + input;
}
}
Step 3: Start the RMI Registry and Bind the Service
import java.rmi.registry.LocateRegistry;
import java.rmi.registry.Registry;
public class ServerMain {
public static void main(String[] args) {
try {
MethodServer server = new MethodServerImpl();
Registry registry = LocateRegistry.createRegistry(1099);
registry.rebind("MethodServer", server);
System.out.println("Java Method Server is running...");
} catch (Exception e) {
e.printStackTrace();
}
}
}
Step 4: Implement the Client
import java.rmi.registry.LocateRegistry;
import java.rmi.registry.Registry;
public class ClientMain {
public static void main(String[] args) {
try {
Registry registry = LocateRegistry.getRegistry("localhost", 1099);
MethodServer server = (MethodServer) registry.lookup("MethodServer");
String response = server.executeMethod("Hello World");
System.out.println("Response from server: " + response);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Best Practices for Java Method Server
Optimize Method Execution: Ensure methods execute efficiently to avoid performance bottlenecks.
Implement Security Measures: Use authentication and authorization for method access.
Use Load Balancing: Distribute requests effectively to enhance performance.
Monitor Server Performance: Log execution times and errors for optimization.
Ensure Scalability: Design the system to handle increasing workloads effectively.
Conclusion
Java Method Server is an effective solution that allows Java methods to be executed remotely, increasing the scalability and efficiency of applications. The implementation procedures and recommended practices described in this blog can help developers create reliable, distributed applications that make efficient use of server resources.
Recommended Posts:-
Comments